---
title: Everything Be True
---
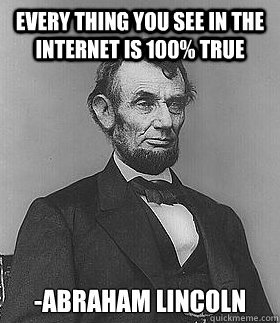
 Remember to use **`Read-Search-Ask`** if you get stuck. Try to pair program  and write your own code 
###  Problem Explanation:
The program needs to check if the second argument is a truthy element, and it must check this for each object in the first argument.
#### Relevant Links
* JavaScript Truthy
* JavaScript Arguments
##  Hint: 1
Remember to iterate through the first argument to check each object.
> _try to solve the problem now_
##  Hint: 2
Only if all of them are truth will we return true, so make sure all of them check.
> _try to solve the problem now_
##  Hint: 3
> _try to solve the problem now_
You could use loops or callbacks functions, there are multiple ways to solve this problem.
## Spoiler Alert!
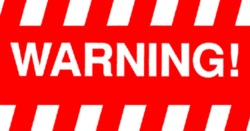
**Solutions ahead!**
##  Basic Code Solution:
**Using for-in loop & hasOwnProperty**
function truthCheck(collection, pre) {
// Create a counter to check how many are true.
var counter = 0;
// Check for each object
for (var c in collection) {
// If it is has property and value is truthy
if (collection[c].hasOwnProperty(pre) && Boolean(collection[c][pre])) {
counter++;
}
}
// Outside the loop, check to see if we got true for all of them and return true or false
return counter == collection.length;
}
// test here
truthCheck([{"user": "Tinky-Winky", "sex": "male"}, {"user": "Dipsy", "sex": "male"}, {"user": "Laa-Laa", "sex": "female"}, {"user": "Po", "sex": "female"}], "sex");
 Run Code
### Code Explanation:
* First I create a counter to check how many cases are actually true.
* Then check for each object if the value is truthy
* Outside the loop, I check to see if the counter variable has the same value as the length of **collection**, if true then return **true**, otherwise, return **false**
#### Relevant Links
* JS Loops
* Object.prototype.hasOwnProperty()
##  Intermediate Code Solution:
**Using Array.every()**
function truthCheck(collection, pre) {
return collection.every(function (element) {
return element.hasOwnProperty(pre) && Boolean(element[pre]);
});
}
// test here
truthCheck([{"user": "Tinky-Winky", "sex": "male"}, {"user": "Dipsy", "sex": "male"}, {"user": "Laa-Laa", "sex": "female"}, {"user": "Po", "sex": "female"}], "sex");
 Run Code
### Code Explanation:
* Uses the native "every" method to test whether all elements in the array pass the test.
* This link will help Array.prototype.every()
#### Relevant Links
* JS Array.prototype.every()
* MDN Array.prototype.every()
##  Advanced Code Solution:
function truthCheck(collection, pre) {
// Is everyone being true?
return collection.every(obj => obj[pre]);
}
truthCheck([{"user": "Tinky-Winky", "sex": "male"}, {"user": "Dipsy", "sex": "male"}, {"user": "Laa-Laa", "sex": "female"}, {"user": "Po", "sex": "female"}], "sex");
 Run Code
### Code Explanation:
* For _every_ object in the `collection` array, check the truthiness of object's property passed in `pre` parameter
* `Array#every` method internally checks if the value returned from the callback is truthy.
* Return `true` if it passes for _every_ object. Otherwise, return `false`.
#### Relevant Links
* `Array#every`
##  NOTES FOR CONTRIBUTIONS:
*  **DO NOT** add solutions that are similar to any existing solutions. If you think it is **_similar but better_**, then try to merge (or replace) the existing similar solution.
* Add an explanation of your solution.
* Categorize the solution in one of the following categories — **Basic**, **Intermediate** and **Advanced**. 
* Please add your username only if you have added any **relevant main contents**. ( **_DO NOT_** _remove any existing usernames_)
> See  **`Wiki Challenge Solution Template`** for reference.